Audio Processing#
[1]:
import numpy as np
import matplotlib.pyplot as plt
from matplotlib import style
style.use('ggplot')
Setup pyaudio#
[2]:
import pyaudio
CHUNK_SIZE = 1024 * 4 # how many audio samples per frame
FORMAT = pyaudio.paInt16 # 16 bit audio format in bits
CHANNELS = 1
RATE = 44100 # samples per second : 44.1 KHz
# Port audio interface on Pyaudio
p = pyaudio.PyAudio()
Setup stream#
[3]:
stream = p.open(
format=FORMAT,
channels=CHANNELS,
rate=RATE,
input=True,
output=True,
frames_per_buffer=CHUNK_SIZE
)
Read stream#
[4]:
bit_data = stream.read(CHUNK_SIZE) #this data is in bits. and python will display it in the hex format
print(bit_data)
b"\\\x00S\x00X\x00Q\x00S\x00Q\x00G\x00Q\x00E\x00J\x00P\x00N\x00\\\x00Z\x00H\x008\x00G\x00G\x00@\x00.\x00:\x008\x008\x003\x00>\x00*\x00*\x00'\x008\x00:\x00>\x007\x003\x001\x00'\x00!\x00'\x00%\x00!\x00%\x00\x11\x00\x10\x00\x18\x00\x13\x00#\x00.\x00\x1e\x00\x15\x00\x18\x00 \x00\x18\x00\x18\x00\n\x00\x15\x00\x18\x00,\x00\x1c\x00(\x00,\x001\x00.\x00@\x00:\x00A\x007\x00*\x00,\x000\x001\x00.\x00#\x00H\x00C\x00*\x00G\x00S\x00Q\x00C\x00A\x000\x00@\x007\x000\x00:\x00%\x001\x005\x00,\x00%\x00,\x00 \x00'\x003\x00#\x000\x00\x17\x00\x08\x00\x18\x00\x15\x00\x18\x00\x10\x00\x15\x00\x10\x00\x0e\x00\x1a\x00\n\x00\xf8\xff\x13\x00\x07\x00\x03\x00\x10\x00\x0e\x00\x15\x00\x15\x00\x10\x00%\x00\x13\x00 \x00(\x00,\x00(\x00>\x00G\x00#\x00!\x00!\x007\x001\x007\x00:\x00.\x00#\x00\x1c\x00\n\x00\x18\x00\x13\x00\n\x00\x05\x00\xfa\xff\x05\x00\xf7\xff\xf8\xff\xf7\xff\xee\xff\x01\x00\xfc\xff\xf5\xff\xee\xff\xf1\xff\xef\xff\xf3\xff\x01\x00\xfc\xff\xfa\xff\xf5\xff\x01\x00\xfa\xff\xfe\xff\xea\xff\xf7\xff\x03\x00\xfc\xff\xf8\xff\n\x00\x08\x00\x07\x00\x07\x00\x03\x00\x05\x00\xfa\xff\x13\x00\x1a\x00\x13\x00\x1e\x00\x15\x00\x15\x00\x17\x00\x17\x00 \x00\x10\x00\x11\x00\x1c\x00\x17\x00\x1e\x00*\x00%\x00%\x00!\x00(\x00\x1e\x00\x0e\x00\x07\x00\n\x00\x0c\x00\x03\x00\x05\x00\x07\x00\x03\x00\x00\x00\x03\x00\x07\x00\x07\x00\x0e\x00\x15\x00\x07\x00\x15\x00!\x00\x1c\x00\x13\x00\x17\x00\x13\x00\x08\x00\x1a\x00\x10\x00\x1c\x00\x11\x00\x17\x00\x0e\x00\x11\x00\x10\x00\x05\x00\x05\x00\x07\x00\x01\x00\x0e\x00\x07\x00\n\x00\xfa\xff\xef\xff\xf5\xff\xf7\xff\x03\x00\xfc\xff\x01\x00\x07\x00\xf7\xff\xfa\xff\x01\x00\xf8\xff\xf1\xff\xf7\xff\xf3\xff\xf8\xff\xef\xff\xe8\xff\xf1\xff\xf5\xff\x01\x00\xf5\xff\xfc\xff\xf3\xff\xf8\xff\xee\xff\xf8\xff\xf3\xff\xf5\xff\xe7\xff\xda\xff\xd8\xff\xe8\xff\xf1\xff\xf7\xff\xf7\xff\xec\xff\xe8\xff\xee\xff\xf1\xff\x01\x00\xf3\xff\xfa\xff\xfc\xff\x01\x00\x01\x00\x08\x00\xf8\xff\xe7\xff\x00\x00\xf8\xff\x00\x00\xf8\xff\xf8\xff\xea\xff\xf1\xff\xea\xff\xec\xff\xe3\xff\xea\xff\xee\xff\xea\xff\xea\xff\xe1\xff\xe1\xff\xe3\xff\xee\xff\xe7\xff\xea\xff\x03\x00\xd5\xff\xde\xff\xdc\xff\xe5\xff\xe1\xff\xec\xff\xef\xff\xe1\xff\xdf\xff\xea\xff\xe7\xff\xe8\xff\xde\xff\xd3\xff\xea\xff\xf7\xff\xf5\xff\xe1\xff\xfa\xff\xfe\xff\xfc\xff\x00\x00\x00\x00\x03\x00\xfa\xff\xf7\xff\xee\xff\xf5\xff\xe5\xff\xe7\xff\xea\xff\xe3\xff\xde\xff\xd7\xff\xcf\xff\xce\xff\xcf\xff\xbc\xff\xc5\xff\xc8\xff\xd1\xff\xce\xff\xc1\xff\xc7\xff\xc3\xff\xbc\xff\xbe\xff\xbe\xff\xb7\xff\xbf\xff\xbe\xff\xbf\xff\xb5\xff\xa8\xff\xb1\xff\xa8\xff\xbe\xff\xaf\xff\xaf\xff\xa7\xff\xaa\xff\xa5\xff\xa7\xff\x9c\xff\xa5\xff\xaa\xff\xa8\xff\xa7\xff\x9f\xff\xa7\xff\x9a\xff\xa7\xff\xb1\xff\xaf\xff\xac\xff\x9e\xff\xa7\xff\xa3\xff\xae\xff\xaa\xff\xb8\xff\xba\xff\xb8\xff\xc3\xff\xbe\xff\xc5\xff\xb3\xff\xb8\xff\xb5\xff\xb8\xff\xb8\xff\xc3\xff\xc3\xff\xbf\xff\xce\xff\xc7\xff\xbf\xff\xbf\xff\xca\xff\xd3\xff\xce\xff\xc7\xff\xcc\xff\xc7\xff\xbf\xff\xcc\xff\xbe\xff\xc1\xff\xbf\xff\xb3\xff\xb5\xff\xb5\xff\xb5\xff\xc1\xff\xd1\xff\xc5\xff\xc1\xff\xaf\xff\xc3\xff\xb7\xff\xb5\xff\xb1\xff\xa7\xff\x95\xff\x9e\xff\xa5\xff\xa8\xff\xa7\xff\x9e\xff\xaf\xff\xa5\xff\xac\xff\xa8\xff\x9a\xff\x8e\xff\x91\xff\x98\xff\x8f\xff\x93\xff\x9a\xff\x9a\xff\x97\xff\x91\xff\x98\xff\x9e\xff\x9a\xff\x8a\xff\x85\xff\x83\xff\x81\xff\x81\xff\x8a\xff\x95\xff\x93\xff\x9f\xff\x9c\xff\xa5\xff\x9f\xff\xa7\xff\x9c\xff\xb8\xff\xd5\xff\xca\xff\xba\xff\xc8\xff\xcc\xff\xd5\xff\xe3\xff\xce\xff\xd3\xff\xd8\xff\xd1\xff\xc5\xff\xb7\xff\xaf\xff\xac\xff\xaf\xff\xaf\xff\x9e\xff\xa7\xff\xb1\xff\xa5\xff\xac\xff\xb3\xff\xb3\xff\xbe\xff\xbe\xff\xae\xff\xba\xff\xb7\xff\xac\xff\xb3\xff\xb5\xff\xae\xff\xa7\xff\xb1\xff\xb7\xff\xbc\xff\xba\xff\xb3\xff\xaa\xff\xa1\xff\xbc\xff\xac\xff\xa8\xff\x91\xff\x9f\xff\x9c\xff\xa3\xff\x93\xff\x9e\xff\x98\xff\x9c\xff\x9a\xff\x97\xff\x95\xff\x9c\xff\x9a\xff\x9e\xff\x9c\xff\x9f\xff\xac\xff\xae\xff\xac\xff\x9e\xff\xac\xff\xa1\xff\x9a\xff\x95\xff\x9c\xff\x91\xff\x8c\xff\x87\xff\x87\xff\x8e\xff\x93\xff\x95\xff\xa7\xff\xa5\xff\xa5\xff\x91\xff\x9f\xff\xa8\xff\xae\xff\xae\xff\xb3\xff\xa8\xff\xac\xff\xac\xff\xae\xff\xb3\xff\xb5\xff\xbc\xff\xb7\xff\xb5\xff\xa8\xff\xbc\xff\xba\xff\xc8\xff\xca\xff\xc5\xff\xc1\xff\xc1\xff\xb8\xff\xba\xff\xb8\xff\xb7\xff\xaf\xff\xa5\xff\x9a\xff\xac\xff\xb8\xff\xb5\xff\xa8\xff\xa8\xff\xb3\xff\xae\xff\xa8\xff\xa8\xff\xb8\xff\xb5\xff\xae\xff\xaa\xff\xa7\xff\xb5\xff\xaa\xff\xb5\xff\xaf\xff\xac\xff\xba\xff\xb7\xff\xbf\xff\xc8\xff\xbf\xff\xb7\xff\xbc\xff\xbc\xff\xca\xff\xcf\xff\xdc\xff\xda\xff\xd7\xff\xe3\xff\xe5\xff\xea\xff\xe8\xff\xe8\xff\xdc\xff\xe8\xff\xe8\xff\xf1\xff\xef\xff\xde\xff\xd5\xff\xe8\xff\xea\xff\xd3\xff\xda\xff\xe1\xff\xe5\xff\xe1\xff\xe7\xff\xe5\xff\xda\xff\xda\xff\xde\xff\xd8\xff\xca\xff\xc3\xff\xbc\xff\xbe\xff\xba\xff\xba\xff\xb3\xff\xae\xff\xb1\xff\xc8\xff\xba\xff\xaa\xff\xbc\xff\xae\xff\xb1\xff\xa7\xff\x9c\xff\xa7\xff\xaf\xff\xa8\xff\xae\xff\xb5\xff\xba\xff\xae\xff\xb5\xff\xbe\xff\xce\xff\xd8\xff\xcc\xff\xd3\xff\xc8\xff\xc8\xff\xc8\xff\xcf\xff\xd7\xff\xce\xff\xbc\xff\xae\xff\xbf\xff\xbc\xff\xc3\xff\xba\xff\xb5\xff\xb5\xff\xae\xff\xb1\xff\xae\xff\xa1\xff\xba\xff\xce\xff\xc7\xff\xd1\xff\xb8\xff\xbe\xff\xc1\xff\xca\xff\xc3\xff\xc5\xff\xbf\xff\xb3\xff\xce\xff\xc5\xff\xb5\xff\xc5\xff\xe1\xff\xdc\xff\xe8\xff\xee\xff\x01\x00\x07\x00\xf7\xff\x10\x00\x01\x00\x10\x00\x0c\x00\x15\x00\x18\x00\x1c\x00#\x00\x11\x00\x07\x00\x10\x00\x08\x00\x10\x00\x05\x00\x03\x00\x03\x00\x05\x00\x08\x00\x10\x00\xfe\xff\x03\x00\xf8\xff\xf8\xff\xfe\xff\xfa\xff\xee\xff\xfc\xff\xf8\xff\xf7\xff\xef\xff\xe5\xff\xe7\xff\xee\xff\xe5\xff\xdc\xff\xdf\xff\xc5\xff\xca\xff\xd3\xff\xe1\xff\xe7\xff\xea\xff\xf7\xff\xf8\xff\xf7\xff\xd8\xff\xe7\xff\xf5\xff\xea\xff\xec\xff\xea\xff\xee\xff\xec\xff\xef\xff\xec\xff\xf7\xff\xec\xff\xe5\xff\xe7\xff\xe5\xff\xfe\xff\xf7\xff\xec\xff\xe7\xff\xdf\xff\xe5\xff\xe7\xff\xe1\xff\xee\xff\xf7\xff\xf3\xff\x05\x00\x03\x00\x05\x00\x03\x00\x00\x00\x07\x00\x07\x00\x07\x00\xfc\xff\x00\x00\xfe\xff\xf5\xff\x01\x00\xfc\xff\xfc\xff\xef\xff\xf8\xff\xf3\xff\xef\xff\xfe\xff\x05\x00\x08\x00\x10\x00\x0c\x00\x0c\x00\x07\x00\x10\x00\x10\x00\n\x00\x03\x00\xfc\xff\xfe\xff\x07\x00\x07\x00\xef\xff\xf5\xff\xe5\xff\xf5\xff\xf7\xff\x01\x00\x00\x00\x11\x00\xf7\xff\xef\xff\xf8\xff\x00\x00\x01\x00\xf8\xff\x07\x00\xfa\xff\xf7\xff\xee\xff\xde\xff\xea\xff\xd8\xff\xd1\xff\xd3\xff\xd5\xff\xd5\xff\xc7\xff\xc5\xff\xca\xff\xd8\xff\xc5\xff\xd1\xff\xc8\xff\xd5\xff\xc7\xff\xce\xff\xce\xff\xcf\xff\xd5\xff\xcf\xff\xe1\xff\xdc\xff\xce\xff\xce\xff\xd1\xff\xc5\xff\xc5\xff\xc8\xff\xc1\xff\xc8\xff\xbc\xff\xba\xff\xbe\xff\xbf\xff\xb1\xff\xaf\xff\xc5\xff\xb8\xff\xc1\xff\xbe\xff\xdf\xff\xca\xff\xcf\xff\xbe\xff\xcf\xff\xc7\xff\xc1\xff\xc8\xff\xbf\xff\xc7\xff\xbc\xff\xb5\xff\xc8\xff\xd1\xff\xbf\xff\xc7\xff\xc8\xff\xc5\xff\xc3\xff\xb8\xff\xba\xff\xc7\xff\xc5\xff\xc7\xff\xc8\xff\xc3\xff\xd8\xff\xb5\xff\xb3\xff\xaa\xff\xa5\xff\xb1\xff\xaf\xff\xcf\xff\xbc\xff\xc1\xff\xac\xff\xaa\xff\xb1\xff\xae\xff\xa5\xff\x9c\xff\x98\xff\x97\xff\x95\xff\x9a\xff\x9c\xff\xb5\xff\xac\xff\xa3\xff\xa7\xff\x97\xff\xa7\xff\x98\xff\xaa\xff\xac\xff\xb5\xff\xae\xff\xa5\xff\xb7\xff\x9e\xff\xb7\xff\xac\xff\x9e\xff\xb1\xff\xb1\xff\x9e\xff\xa7\xff\xaa\xff\xa7\xff\x9e\xff\x91\xff\x93\xff\x8e\xff\x87\xff\x87\xff\x8c\xff\x8a\xff\x8c\xff\x8c\xff\x8a\xff\x93\xff\x93\xff\x9c\xff\x9f\xff\xa3\xff\xa5\xff\xa3\xff\xaa\xff\xaa\xff\xb5\xff\xb1\xff\xa8\xff\xa7\xff\xae\xff\xb1\xff\xb8\xff\xba\xff\xb8\xff\xc1\xff\xcc\xff\xc1\xff\xba\xff\xb1\xff\xb1\xff\xaf\xff\xaa\xff\xaa\xff\xa8\xff\xaa\xff\xa1\xff\xb1\xff\xa3\xff\x9c\xff\x9a\xff\x9c\xff\xa3\xff\xaa\xff\xac\xff\xa5\xff\x9c\xff\xa8\xff\xa7\xff\x9a\xff\x9e\xff\xa7\xff\xa1\xff\xae\xff\xa8\xff\xac\xff\x9c\xff\xa3\xff\xa1\xff\xa1\xff\x93\xff\x97\xff\x97\xff\x95\xff\x8f\xff~\xffw\xff\x7f\xff\x9c\xff\x9f\xff\xa3\xff\x9e\xff\xaa\xff\x9c\xff\x9e\xff\x8f\xff\x93\xff\x97\xff\xa5\xff\xa1\xff\xa5\xff\x9a\xff\xa3\xff\x9f\xff\x8e\xff\x88\xff\x85\xff\x9f\xff\x9c\xff\x9a\xff\x8c\xff\x88\xff\x95\xff\x97\xff\x9f\xff\xa5\xff\xaf\xff\xb1\xff\xb1\xff\xb8\xff\xb3\xff\xb3\xff\xa7\xff\xa1\xff\x9a\xff\xa8\xff\xa5\xff\xaa\xff\xa3\xff\xa7\xff\xaa\xff\xb7\xff\xb1\xff\xaa\xff\xae\xff\xa3\xff\xb5\xff\xaf\xff\xaf\xff\xb1\xff\xb7\xff\xb1\xff\xb3\xff\xb5\xff\xb5\xff\xbc\xff\xc3\xff\xba\xff\xc3\xff\xb8\xff\xae\xff\xae\xff\xb8\xff\xb1\xff\xaa\xff\xb1\xff\xb5\xff\xb5\xff\xac\xff\xaa\xff\xa7\xff\xb1\xff\xb8\xff\xc3\xff\xbe\xff\xc5\xff\xce\xff\xc1\xff\xbe\xff\xc1\xff\xc1\xff\xac\xff\xa3\xff\xbe\xff\xbe\xff\xbe\xff\xc7\xff\xbe\xff\xc3\xff\xce\xff\xbf\xff\xbc\xff\xd3\xff\xca\xff\xcc\xff\xc5\xff\xce\xff\xbc\xff\xb5\xff\xbe\xff\xba\xff\xbc\xff\xbc\xff\xb8\xff\xbf\xff\xb7\xff\xaa\xff\xc7\xff\xc3\xff\xc1\xff\xaf\xff\xaf\xff\xb5\xff\xac\xff\xb7\xff\xb5\xff\xaf\xff\xa5\xff\x9c\xff\x9f\xff\x9f\xff\x8f\xff\x81\xff\x95\xff\x95\xff\x9f\xff\xa1\xff\xaa\xff\xa5\xff\xac\xff\xa8\xff\xb1\xff\xaa\xff\xaa\xff\xba\xff\xb7\xff\xa3\xff\xae\xff\xa5\xff\xb5\xff\xbc\xff\xc1\xff\xc8\xff\xbe\xff\xbe\xff\xc5\xff\xb8\xff\xc8\xff\xc5\xff\x9e\xff\xa7\xff\xa3\xff\xa8\xff\xbe\xff\x98\xff\x9c\xff\xac\xff\x9f\xff\xa1\xff\x85\xff\x93\xff\x88\xff\x9c\xff\x9f\xff\x8e\xff\x95\xff\x91\xff\x93\xff\x9a\xff\xb7\xff\xa3\xff\xae\xff\xba\xff\xc5\xff\x9e\xff\xac\xff\xb1\xff\xae\xff\xa3\xff\xa3\xff\x95\xff\xa5\xff\xba\xff\xb5\xff\xa5\xff\xac\xff\xa5\xff\xa8\xff\x8e\xff\x9f\xff\xa8\xff\xa7\xff\xa3\xff\xa7\xff\xb3\xff\x8f\xff\xa7\xff\xa1\xff\xa1\xff\x9c\xff\xa1\xff\xa1\xff\x95\xff\x97\xff\x8f\xff\x9f\xff\xb3\xff\xbf\xff\xb5\xff\xa5\xff\xac\xff\x98\xff\xac\xff\xbe\xff\xae\xff\x9e\xff\x9c\xff\x98\xff\xa1\xff\xa8\xff\x9c\xff\xb1\xff\xb3\xff\xb5\xff\xa5\xff\xa5\xff\x97\xff\x91\xff\x93\xff\xa3\xff\x9a\xff\xaa\xff\xa3\xff\xb1\xff\xb8\xff\xbf\xff\xa3\xff\xa8\xff\xa7\xff\xac\xff\xae\xff\xa5\xff\x9e\xff\xa1\xff\xae\xff\xaa\xff\x9f\xff\xa1\xff\xaa\xff\xae\xff\xa8\xff\xae\xff\xba\xff\xbe\xff\xce\xff\xc3\xff\xcc\xff\xae\xff\xac\xff\xae\xff\xb8\xff\xaf\xff\xc1\xff\xb3\xff\xb1\xff\xb5\xff\xc7\xff\xca\xff\xb5\xff\xbc\xff\xbc\xff\xba\xff\xb1\xff\xaf\xff\xb1\xff\xa3\xff\x9c\xff\xa7\xff\xac\xff\xb3\xff\xa7\xff\xb5\xff\xb7\xff\xe7\xff\xd1\xff\xb8\xff\xca\xff\xd5\xff\xd8\xff\xd7\xff\xd8\xff\xc8\xff\xbc\xff\xc1\xff\xb7\xff\xba\xff\xa8\xff\xc5\xff\xc3\xff\xbc\xff\xca\xff\xc5\xff\xc3\xff\xcc\xff\xd1\xff\xcc\xff\xce\xff\xc3\xff\xc3\xff\xd1\xff\xd1\xff\xd1\xff\xd7\xff\xcc\xff\xc1\xff\xd3\xff\xd8\xff\xd8\xff\xd3\xff\xd8\xff\xcc\xff\xd1\xff\xca\xff\xca\xff\xbe\xff\xbf\xff\xcf\xff\xc3\xff\xb7\xff\xa7\xff\xb1\xff\xb3\xff\xb8\xff\xc1\xff\xbe\xff\xba\xff\xa7\xff\xc1\xff\xbc\xff\xaf\xff\xbf\xff\xc8\xff\xc3\xff\xca\xff\xce\xff\xc1\xff\xca\xff\xbf\xff\xb5\xff\xb8\xff\xc5\xff\xba\xff\xc5\xff\xcf\xff\xc8\xff\xc3\xff\xbc\xff\xc3\xff\xd1\xff\xce\xff\xbf\xff\xba\xff\xc5\xff\xd5\xff\xd1\xff\xcf\xff\xcc\xff\xc7\xff\xc1\xff\xcc\xff\xcc\xff\xcc\xff\xc7\xff\xca\xff\xc7\xff\xbe\xff\xa5\xff\xaf\xff\xa3\xff\xbc\xff\xa5\xff\xa1\xff\xaf\xff\xbe\xff\xaf\xff\xb1\xff\xb5\xff\xba\xff\xb8\xff\xb5\xff\xc3\xff\xa8\xff\x9f\xff\xae\xff\xba\xff\xb1\xff\xaf\xff\xbe\xff\xb5\xff\xc8\xff\xd7\xff\xd3\xff\xd3\xff\xd5\xff\xe1\xff\xd5\xff\xea\xff\xe7\xff\xe8\xff\xe8\xff\xea\xff\xef\xff\xef\xff\xe5\xff\xf1\xff\xee\xff\xe5\xff\xe8\xff\xda\xff\xcf\xff\xd3\xff\xcf\xff\xd7\xff\xf1\xff\xce\xff\xc8\xff\xbe\xff\xcf\xff\xd1\xff\xd5\xff\xda\xff\xe5\xff\xe7\xff\xf5\xff\xf8\xff\xf1\xff\xf3\xff\xe7\xff\xe3\xff\xe8\xff\xe8\xff\xe5\xff\xd7\xff\xe1\xff\xde\xff\xd7\xff\xd1\xff\xe1\xff\xda\xff\xd5\xff\xf5\xff\xe8\xff\xe7\xff\xe7\xff\xf1\xff\xf8\xff\xf1\xff\xee\xff\xf1\xff\xe7\xff\xee\xff\xf7\xff\x00\x00\xfc\xff\xfe\xff\x11\x00\x11\x00\x03\x00\xfe\xff\x03\x00\xf8\xff\x07\x00\x01\x00\x1e\x00\x18\x00\x17\x00\x0c\x00\n\x00\x08\x00\x08\x00\x07\x00\x07\x00\x10\x00\n\x00\x0c\x00\x05\x00\x01\x00\x01\x00\xf8\xff\x13\x00\x0c\x00\x11\x00\n\x00\x17\x00\x07\x00\xec\xff\xf5\xff\xfa\xff\xfc\xff\xf8\xff\x00\x00\xf7\xff\x0e\x00\x05\x00\xfa\xff\x05\x00\x17\x00 \x00\x0e\x00\x08\x00\x10\x00\n\x00\x0e\x00\x17\x00\x18\x00\x05\x00\x07\x00\x10\x00\x15\x00\n\x00\x08\x00\x11\x00\x18\x00\x17\x00\x1c\x00 \x00\x18\x00'\x00#\x00 \x00\x1c\x00,\x00.\x003\x00,\x00(\x00#\x00\x1e\x00\x1c\x00\x1c\x00\x1e\x00#\x00\x15\x00\x11\x00\x15\x00\x10\x00\x17\x00\x10\x00\x0e\x00\x1c\x00#\x00\x11\x00\x13\x00\x13\x00\x11\x00\x0e\x00\x0c\x00\x1c\x00\x18\x00\x13\x00\x18\x00\x10\x00'\x000\x00*\x003\x00%\x00#\x00%\x00\x1a\x003\x007\x00'\x00#\x00%\x00,\x00(\x003\x00@\x00(\x00\x15\x00\x17\x00#\x00\x1c\x00!\x001\x00<\x007\x00>\x00@\x001\x007\x005\x007\x00*\x00'\x00!\x00\x1a\x00\x1e\x00 \x00 \x00 \x00\x1c\x00!\x00\x1a\x00\x11\x00!\x00\x1e\x00\x1c\x00\x1c\x00\x13\x00\x17\x00\x0e\x00\x0c\x00\x13\x00\x10\x00\x08\x00\x13\x00\x1c\x00\x1c\x00,\x00,\x000\x00#\x00 \x00\x1e\x00*\x00(\x00\x15\x00\x1c\x00\x13\x00\x13\x00\x1e\x00\x1e\x00\x13\x00\x11\x00\x15\x00\x1e\x00\x11\x00\x13\x00\x13\x00\x0e\x00\x01\x00\x07\x00\x0e\x00\x0c\x00\x05\x00\xf8\xff\x01\x00\x01\x00\xf8\xff\xe7\xff\xe7\xff\xf1\xff\x01\x00\xf3\xff\xef\xff\xf5\xff\xf3\xff\xe1\xff\xea\xff\xe1\xff\xec\xff\xe5\xff\xec\xff\xf1\xff\xf1\xff\xf5\xff\xf5\xff\xf5\xff\xf3\xff\xf1\xff\xf8\xff\xf7\xff\xf8\xff\xfe\xff\xf8\xff\xfe\xff\xf5\xff\xef\xff\xec\xff\xec\xff\xe3\xff\xea\xff\xf7\xff\x03\x00\x10\x00\x0c\x00\x03\x00\n\x00\x17\x00\n\x00\xfc\xff\xf7\xff\xfe\xff\xe5\xff\xea\xff\xea\xff\xfa\xff\xec\xff\xf8\xff\xf3\xff\xec\xff\xf1\xff\xf1\xff\xdf\xff\xf7\xff\xfe\xff\x10\x00\x07\x00\x07\x00\x13\x00\x17\x00\x0e\x00\x11\x00\x13\x00\x11\x00\x10\x00\x08\x00\x08\x00\x07\x00\xee\xff\xef\xff\xf3\xff\x00\x00\x08\x00\x07\x00\n\x00\x0e\x00\n\x00\x08\x00\x01\x00\x03\x00\x11\x00\x07\x00\x07\x00\n\x00\x17\x00\x13\x00\x13\x00\n\x00\x07\x00\x0e\x00\x13\x00\n\x00\x03\x00\x07\x00\x05\x00\x08\x00\x08\x00\x01\x00\x00\x00\x08\x00\x0e\x00\x10\x00\xfe\xff\x05\x00\x00\x00\x03\x00\xfe\xff\x00\x00\xfe\xff\x01\x00\x05\x00\x07\x00\xf1\xff\xfa\xff\xef\xff\xe7\xff\xea\xff\xef\xff\xe8\xff\xec\xff\x03\x00\xf3\xff\xf8\xff\xf5\xff\xea\xff\xd3\xff\xe3\xff\xf7\xff\xfe\xff\xea\xff\xef\xff\xf7\xff\x00\x00\xf7\xff\xf1\xff\xf1\xff\xf8\xff\xfc\xff\x01\x00\xe7\xff\xec\xff\xfa\xff\xf8\xff\x01\x00\xf3\xff\xfc\xff\xf7\xff\xf1\xff\xe1\xff\xda\xff\xe3\xff\xcf\xff\xc7\xff\xd7\xff\xda\xff\xdc\xff\xdf\xff\xe1\xff\xd1\xff\xbe\xff\xbe\xff\xb8\xff\xbe\xff\xc7\xff\xc3\xff\xd1\xff\xd3\xff\xdf\xff\xda\xff\xde\xff\xea\xff\xe5\xff\xdf\xff\xde\xff\xf5\xff\xee\xff\xf3\xff\xe5\xff\xea\xff\xec\xff\xe8\xff\xe8\xff\xec\xff\xe8\xff\xdf\xff\xea\xff\xf3\xff\xfe\xff\xf3\xff\xfc\xff\xe5\xff\xf3\xff\xec\xff\xf8\xff\x01\x00\x01\x00\x05\x00\xf8\xff\x00\x00\x01\x00\xf8\xff\xee\xff\xdf\xff\xe3\xff\xe1\xff\xda\xff\xda\xff\xe3\xff\xd8\xff\xd7\xff\xd5\xff\xd8\xff\xdc\xff\xda\xff\xda\xff\xdc\xff\xd8\xff\xda\xff\xdf\xff\xee\xff\xee\xff\xe8\xff\xe7\xff\xd7\xff\xd8\xff\xd3\xff\xdf\xff\xd1\xff\xdf\xff\xd5\xff\xc8\xff\xc3\xff\xb8\xff\xb7\xff\xcf\xff\xc1\xff\xc1\xff\xb8\xff\xb7\xff\xb7\xff\xb1\xff\xbc\xff\xbe\xff\xb8\xff\xa3\xff\xae\xff\xac\xff\xba\xff\xc3\xff\xc5\xff\xbe\xff\xb8\xff\xbc\xff\xca\xff\xc7\xff\xc8\xff\xba\xff\xcf\xff\xc5\xff\xd5\xff\xc8\xff\xcc\xff\xbe\xff\xc7\xff\xae\xff\xbc\xff\xc3\xff\xc1\xff\xbe\xff\xb8\xff\xbf\xff\xc8\xff\xd1\xff\xd3\xff\xd7\xff\xcc\xff\xd3\xff\xd3\xff\xde\xff\xde\xff\xec\xff\xda\xff\xe8\xff\xd7\xff\xec\xff\xee\xff\xf5\xff\xec\xff\xf8\xff\xf7\xff\n\x00\x01\x00\xfe\xff\x00\x00\x03\x00\x0c\x00\x0c\x00\x17\x00\x10\x00\n\x00\x03\x00\x07\x00\xfa\xff\x03\x00\xfa\xff\xf1\xff\xe1\xff\xe1\xff\xe1\xff\xee\xff\xdf\xff\xec\xff\xe8\xff\xf7\xff\xee\xff\xec\xff\xec\xff\xfe\xff\x03\x00\x05\x00\x0c\x00\x0e\x00\x0c\x00\x0c\x00\x18\x00\x0c\x00\x1c\x00\x11\x00'\x00\x1a\x00\x17\x00\x0e\x00\x0c\x00\x07\x00\n\x00\x15\x00\x11\x00\x15\x00\x17\x00\x1c\x00\x1e\x00\x17\x00\x18\x00\x1a\x00(\x00%\x00\x1c\x00\x1e\x00%\x00\x17\x00\x17\x00!\x00\x1e\x00\x17\x00\n\x00\x1c\x00\x15\x00\x17\x00\x17\x00%\x00\x1c\x00\x11\x00\x15\x00\x1e\x00\x17\x00\x1a\x00\x10\x00\x10\x00\x1c\x00\x10\x00#\x00%\x00\x1c\x00\x0e\x00\x13\x00\x1a\x00\x15\x00!\x00\x17\x00\x1a\x00\x15\x00\x17\x00\x1e\x00 \x00,\x00%\x00(\x00(\x00#\x00!\x00.\x001\x003\x003\x00,\x00:\x00>\x00!\x00\x15\x00 \x00\x11\x00\x08\x00\x0e\x00\x08\x00\xfa\xff\x08\x00\x03\x00\x0e\x00\x05\x00\x0e\x00\x00\x00\x03\x00\x05\x00\x07\x00\x10\x00\x0c\x00\x10\x00\x0c\x00\x05\x00\x08\x00\x1a\x00\x18\x00\x1e\x00\x0c\x00\x0c\x00\xfa\xff\x08\x00\n\x00\x0c\x00\x07\x00\n\x00\xf7\xff\x00\x00\xf8\xff\xfa\xff\xfa\xff\x01\x00\x05\x00\x0c\x00\x10\x00\n\x00\x0e\x00\xf5\xff\xf8\xff\x03\x00\x0c\x00\x00\x00\x0c\x00\x01\x00\x05\x00\x15\x00\x0c\x00\x03\x00\xfc\xff\xf7\xff\x00\x00\xfe\xff\xf5\xff\xfc\xff\x05\x00\xe3\xff\xf7\xff\xe5\xff\xe7\xff\xdf\xff\xec\xff\xfc\xff\xfa\xff\x00\x00\xfc\xff\xfa\xff\xfa\xff\xf5\xff\xfe\xff\x05\x00\n\x00\x07\x00\x00\x00\x13\x00\xfa\xff\x0e\x00\x13\x00\x10\x00\x1c\x00\x10\x00\x07\x00\x0c\x00\x01\x00\x0c\x00\x17\x00\x11\x00\x1a\x00\x07\x00\n\x00\x13\x00\n\x00\x15\x00\x11\x00\xfa\xff\x11\x00\x13\x00\n\x00\xfc\xff\n\x00\x05\x00\x05\x00\x0e\x00\x05\x00\x10\x00\x05\x00\x1a\x00\x15\x00\x18\x00*\x00*\x00\n\x00\x0e\x00'\x00\x1e\x00\x00\x00\x05\x00\n\x00\x1a\x00\x0e\x00\x10\x00\x17\x00'\x00\x08\x00\x18\x00\x0e\x00 \x00\x1c\x00'\x00,\x00'\x005\x00\x17\x00!\x00.\x000\x00,\x00*\x00.\x00%\x00#\x00\x1c\x00%\x00!\x00\x08\x00\x0c\x00\x08\x00\x15\x00\x11\x00\x13\x00\n\x00\x0e\x00\x17\x00\x17\x00\x0c\x00\x17\x00\x07\x00\x01\x00\xec\xff\xfe\xff\x08\x00\xee\xff\xec\xff\xf3\xff\xdc\xff\xe7\xff\xec\xff\xef\xff\xf1\xff\xfc\xff\xfa\xff\xdf\xff\x01\x00\x00\x00\xfc\xff\x00\x00\x00\x00\xfa\xff\x07\x00\x07\x00\x1c\x00!\x00(\x00\x1c\x00\x1a\x00#\x00 \x00%\x00\x17\x00%\x00'\x00\x1e\x00\x18\x00(\x00\x1a\x00\x15\x00\x17\x00\x0e\x00\x11\x00\x15\x00\x08\x00\x05\x00\n\x00\x1c\x00\x11\x00\x13\x00\x1a\x00#\x00\x10\x00\x11\x00\x0c\x00\x08\x00\x05\x00\x08\x00\x03\x00\x0c\x00\x11\x00\x11\x00\x15\x00\x10\x00\x13\x00\x0e\x00\x07\x00\x08\x00\x11\x00\x15\x00\x17\x00\x15\x00!\x00\x1e\x00\x1c\x00\x0e\x00\n\x00\n\x00\x13\x00\x0e\x00\x11\x00\x0c\x00\x0e\x00\x08\x00\xf5\xff\xef\xff\xf5\xff\xec\xff\xe7\xff\xec\xff\xda\xff\xe3\xff\xe5\xff\xe5\xff\xee\xff\xf3\xff\xe8\xff\xea\xff\xe7\xff\xde\xff\xe5\xff\xef\xff\xea\xff\xf7\xff\xf3\xff\x07\x00\xfc\xff\xd3\xff\xdc\xff\xea\xff\xef\xff\xee\xff\x03\x00\x01\x00\x03\x00\x08\x00\x05\x00\x05\x00\xfc\xff\n\x00\n\x00\x08\x00\xfe\xff\xf5\xff\xfc\xff\xfe\xff\xfc\xff\xf7\xff\xf1\xff\xf1\xff\xd7\xff\xea\xff\xe3\xff\xce\xff\xda\xff\xe7\xff\xe1\xff\xe5\xff\xe7\xff\xee\xff\xf1\xff\xfa\xff\xfc\xff\x00\x00\xf7\xff\xee\xff\xde\xff\xd8\xff\xd3\xff\xce\xff\xcc\xff\xd5\xff\xd1\xff\xcf\xff\xca\xff\xcc\xff\xd3\xff\xd8\xff\xde\xff\xde\xff\xe3\xff\xe7\xff\xdf\xff\xe7\xff\xe7\xff\xde\xff\xe5\xff\xf3\xff\xee\xff\xe5\xff\xf5\xff\xe8\xff\xf5\xff\xf5\xff\xfe\xff\x03\x00\x07\x00\x03\x00\x07\x00\x05\x00\xfe\xff\x05\x00\x0e\x00\x0e\x00\x03\x00\xfc\xff\xef\xff\xf1\xff\xee\xff\xe3\xff\xe5\xff\xdf\xff\xde\xff\xe5\xff\xec\xff\xe8\xff\xe8\xff\xee\xff\xe8\xff\xf1\xff\xef\xff\xf7\xff\xf7\xff\x00\x00\x01\x00\x01\x00\x00\x00\xf8\xff\xe1\xff\xdc\xff\xe5\xff\xe3\xff\xe7\xff\xd7\xff\xdf\xff\xd3\xff\xcc\xff\xd5\xff\xdc\xff\xd1\xff\xd1\xff\xce\xff\xce\xff\xe5\xff\xec\xff\xef\xff\xea\xff\xea\xff\xf1\xff\xf7\xff\xf7\xff\xea\xff\xf7\xff\xe5\xff\xe8\xff\xdf\xff\xc7\xff\xd1\xff\xe3\xff\xd5\xff\xc8\xff\xe5\xff\xd7\xff\xe8\xff\xce\xff\xde\xff\xcc\xff\xd3\xff\xd3\xff\xd7\xff\xce\xff\xce\xff\xc7\xff\xd1\xff\xdf\xff\xe1\xff\xd8\xff\xe7\xff\xf5\xff\xf3\xff\xf1\xff\xe8\xff\xee\xff\xf5\xff\x01\x00\xf5\xff\xfc\xff\xef\xff\xef\xff\xef\xff\xec\xff\xe8\xff\xee\xff\xe5\xff\xd5\xff\xcf\xff\xea\xff\xe8\xff\xea\xff\xec\xff\xea\xff\xef\xff\xf5\xff\xdf\xff\xda\xff\xde\xff\xd8\xff\xda\xff\xd8\xff\xcf\xff\xda\xff\xde\xff\xd8\xff\xde\xff\xdf\xff\xe1\xff\xdf\xff\xe8\xff\xdf\xff\xe1\xff\xd5\xff\xe1\xff\xd3\xff\xd1\xff\xd5\xff\xd7\xff\xde\xff\xe3\xff\xda\xff\xe1\xff\xef\xff\xde\xff\xe5\xff\xd7\xff\xe1\xff\xec\xff\xe5\xff\xdc\xff\xda\xff\xc8\xff\xda\xff\xdc\xff\xde\xff\xce\xff\xc5\xff\xc7\xff\xc5\xff\xc3\xff\xcc\xff\xd5\xff\xc5\xff\xd5\xff\xe3\xff\xdc\xff\xde\xff\xd8\xff\xde\xff\xe1\xff\xe5\xff\xe5\xff\xea\xff\xf5\xff\xf1\xff\xec\xff\xe7\xff\xef\xff\xee\xff\x0c\x00\xfe\xff\x01\x00\x03\x00\x00\x00\xf5\xff\xef\xff\xf3\xff\xee\xff\xe1\xff\xe5\xff\xe8\xff\xe8\xff\xe3\xff\xef\xff\xe5\xff\xe3\xff\xe8\xff\xd8\xff\xda\xff\xda\xff\xcc\xff\xd8\xff\xd3\xff\xd8\xff\xe3\xff\xce\xff\xe5\xff\xd1\xff\xd8\xff\xd7\xff\xe5\xff\xf7\xff\xda\xff\xd7\xff\xdc\xff\xe8\xff\xcf\xff\xe1\xff\xf1\xff\xe1\xff\xde\xff\xde\xff\xee\xff\xd3\xff\xca\xff\xde\xff\xde\xff\xdf\xff\xf5\xff\xee\xff\xde\xff\xe7\xff\xdf\xff\xf3\xff\xfc\xff\xf5\xff\xf5\xff\x01\x00\xf3\xff\xfe\xff\xf8\xff\xf1\xff\xf3\xff\xfc\xff\xf8\xff\xf1\xff\xf8\xff\x00\x00\x11\x00\xfa\xff\x01\x00\xfc\xff\x03\x00\x13\x00\x10\x00\x08\x00\x13\x00\x13\x00\x0c\x00\x1c\x00\x10\x00\x00\x00\xfc\xff\xfa\xff\x05\x00\xfa\xff\xfe\xff\xf7\xff\x03\x00\xfc\xff\x13\x00\x01\x00\xf1\xff\xfa\xff\x0c\x00\x00\x00\xf7\xff\x00\x00\xf8\xff\xf5\xff\xef\xff\xef\xff\xda\xff\xef\xff\xec\xff\xf7\xff\xef\xff\xe3\xff\xec\xff\xee\xff\xee\xff\xf1\xff\xf1\xff\xea\xff\xfe\xff\xef\xff\xf7\xff\xef\xff\x00\x00\xfe\xff\x01\x00\x03\x00\xfc\xff\xfa\xff\xf7\xff\xef\xff\xf5\xff\xf5\xff\xfe\xff\x00\x00\x00\x00\x00\x00\x0e\x00\n\x00\n\x00\x00\x00\xf8\xff\x07\x00\x0c\x00\x17\x00\x10\x00\x0e\x00\xfc\xff\x00\x00\xf8\xff\xfa\xff\xf8\xff\xfa\xff\xf8\xff\xf8\xff\x08\x00\x08\x00\xfe\xff\x01\x00\x07\x00\x0e\x00\x17\x00\x1a\x00\x0c\x00\x1a\x00\x17\x00\x13\x00\x1e\x00*\x00.\x00%\x00\x1c\x00\x1c\x00#\x00\x18\x00\x1e\x00\x18\x00%\x00'\x00,\x00\x18\x00 \x00\x13\x00\x1a\x00\x13\x00\x10\x00\x15\x00\x15\x00\x11\x00!\x00\x17\x00\n\x00\x11\x00\x13\x00\x1a\x00\x18\x00!\x00\x1c\x00(\x00*\x00\x10\x00\x1c\x00!\x00(\x00#\x00'\x00\x1e\x00\x1a\x00\x17\x00\x17\x00\x13\x00!\x00\n\x00\x08\x00\x17\x00\x15\x00\x15\x00\x11\x00\x1c\x00#\x00*\x00\x1c\x00#\x00\x1c\x00\xfc\xff\x11\x00#\x008\x00,\x008\x00\x1e\x001\x00<\x00.\x000\x00\x0c\x00\x17\x00\x1a\x00(\x00\x13\x00\x1a\x00\x10\x00\x15\x00\x18\x00\x18\x00!\x00\n\x00\x13\x00\x10\x00\x11\x00\x05\x00\xfa\xff\xfc\xff\x00\x00\xfe\xff\xf7\xff\xfe\xff\x0c\x00\n\x00\x07\x00\x0e\x00\n\x00\x08\x00\x0c\x00\x17\x00%\x00!\x00(\x00 \x00,\x00*\x00*\x003\x00:\x000\x005\x001\x00*\x00'\x00 \x003\x000\x000\x008\x000\x00>\x00*\x001\x000\x007\x005\x00>\x005\x005\x00*\x00\x1a\x00\x1e\x00 \x00(\x00\x1e\x00\x1e\x00\x1c\x00\x1c\x00\x1a\x00(\x00!\x00\x18\x00\n\x00\x0c\x00\x07\x00\x13\x00#\x00,\x005\x003\x003\x00%\x00#\x00%\x00%\x00!\x00\x15\x00 \x00\x1a\x00 \x00\x11\x00\x1e\x00\x13\x00\x07\x00\x0c\x00\x18\x00\x17\x00\x07\x00\x15\x00\x1c\x00\x10\x00\x07\x00\x17\x00\x15\x00\n\x00\x05\x00\x0e\x00\n\x00\x07\x00\x07\x00\xf7\xff\x08\x00\x01\x00\x03\x00\x08\x00\x15\x00\x18\x00\x17\x00\x10\x00\x0c\x00\x13\x00\x13\x00\x10\x00\x0c\x00\xfe\xff\x07\x00\x0c\x00\x05\x00\xfa\xff\xf5\xff\xee\xff\xfe\xff\x00\x00\x08\x00\x11\x00\n\x00\x11\x00\x08\x00\x13\x00\x03\x00\x13\x00\n\x00\x10\x00\x15\x00\x18\x00\x17\x00\x18\x00!\x00\x1e\x00\x1c\x00%\x00 \x00'\x000\x003\x00\x1e\x00#\x00\x15\x00\x15\x00\x08\x00\x07\x00\x01\x00\xf8\xff\x01\x00\x01\x00\n\x00\x03\x00\x17\x00\x13\x00\x17\x00\n\x00\x15\x00\x07\x00\x13\x00\x01\x00\x0c\x00\x05\x00\x08\x00\x05\x00\x0c\x00\x10\x00\x15\x00\x03\x00\xfe\xff\xf7\xff\xfe\xff\x0e\x00\xf8\xff\xfc\xff\xf1\xff\xf7\xff\xfe\xff\x07\x00\x07\x00\x0e\x00\x01\x00\xf7\xff\xf8\xff\xfa\xff\xea\xff\xee\xff\xe7\xff\xea\xff\xe3\xff\xe5\xff\xe7\xff\xf5\xff\xee\xff\xe5\xff\xd3\xff\xd1\xff\xcf\xff\xce\xff\xd1\xff\xcf\xff\xdc\xff\xde\xff\xec\xff\xe8\xff\xe8\xff\xe7\xff\xe8\xff\xe5\xff\xef\xff\xf5\xff\xe8\xff\xd8\xff\xe3\xff\xd8\xff\xd5\xff\xce\xff\xe3\xff\xe7\xff\xdf\xff\xda\xff\xdc\xff\xcc\xff\xcc\xff\xda\xff\xcc\xff\xd1\xff\xc7\xff\xd3\xff\xc3\xff\xca\xff\xbc\xff\xd3\xff\xca\xff\xcf\xff\xd1\xff\xc8\xff\xe1\xff\xe1\xff\xe3\xff\xe1\xff\xee\xff\xef\xff\xec\xff\xea\xff\xe7\xff\xef\xff\xec\xff\xf5\xff\xec\xff\xe7\xff\xe5\xff\xdc\xff\xe3\xff\xec\xff\x07\x00\xfa\xff\x03\x00\x0c\x00\x1c\x00\x17\x00!\x00\x18\x00!\x00 \x00\n\x00\x08\x00\x08\x00\x05\x00\xfe\xff\xf5\xff\xf8\xff\xfe\xff\xfc\xff\xfa\xff\xe1\xff\xea\xff\xf1\xff\xea\xff\xe5\xff\xe5\xff\xe5\xff\xf8\xff\xee\xff\xf3\xff\xf3\xff\xef\xff\xe7\xff\xf5\xff\xea\xff\xf7\xff\xee\xff\xef\xff\xda\xff\xef\xff\xea\xff\xe8\xff\xee\xff\xdf\xff\xec\xff\xe5\xff\xe1\xff\xd8\xff\xe1\xff\xcf\xff\xe3\xff\xe7\xff\xe8\xff\xf7\xff\xf8\xff\x01\x00\xfe\xff\xfe\xff\xfa\xff\x07\x00\x10\x00\x0c\x00\x1c\x00\x0e\x00\x17\x00\x15\x00\xf8\xff\x11\x00\x08\x00\x10\x00\x10\x00\x0e\x00\x10\x00\x0e\x00\x08\x00\x00\x00\x01\x00\x0e\x00\x13\x00\x1a\x00\x15\x00\x1c\x00'\x00\x18\x00\x11\x007\x00 \x00*\x00'\x00%\x00.\x00\x1c\x00\x1e\x00*\x00*\x00\x08\x00\x03\x00\x05\x00\x0c\x00\n\x00\x10\x00\x07\x00\x0c\x00'\x00\x13\x00\x0c\x00%\x00\x13\x00.\x00\x1a\x00\x11\x00\x18\x00\x08\x00\x0c\x00\x1c\x00#\x00\x18\x00'\x00\x01\x00\x18\x00*\x00,\x00(\x00%\x00'\x00%\x00'\x00,\x00'\x00\x1c\x00%\x00,\x00!\x001\x000\x007\x00E\x007\x00>\x00<\x008\x00:\x00G\x00E\x00>\x00L\x00J\x00A\x001\x00<\x00E\x00G\x00N\x00c\x00Q\x00J\x00e\x00a\x00j\x00c\x00j\x00a\x00a\x00j\x00a\x00X\x00Q\x00@\x00<\x00L\x00P\x00Q\x00`\x00P\x00E\x00C\x00N\x00U\x00W\x00Z\x00`\x00E\x00W\x00Z\x00P\x00E\x00G\x00Q\x00L\x00C\x00G\x00J\x00H\x00N\x00G\x00<\x00<\x003\x00N\x00E\x00G\x00L\x00L\x00H\x00G\x00C\x00E\x00P\x00H\x00<\x00L\x00N\x00J\x00L\x00H\x00>\x00>\x00A\x008\x00@\x00J\x00G\x008\x00A\x00G\x00:\x00<\x00J\x00N\x00G\x00@\x00G\x00:\x00P\x00G\x00J\x00A\x00H\x00A\x00>\x00>\x00<\x00>\x008\x00J\x00A\x00P\x00L\x00Q\x00P\x00g\x00`\x00e\x00`\x00^\x00W\x00J\x00H\x00W\x00a\x00Q\x00S\x00P\x00Z\x00>\x00(\x00<\x003\x00>\x00>\x00@\x00A\x00,\x00 \x00(\x00<\x000\x008\x00C\x00<\x00*\x003\x00*\x00>\x007\x00<\x00>\x003\x00E\x00@\x00G\x00@\x003\x00:\x008\x00:\x000\x003\x007\x00(\x000\x00,\x007\x005\x008\x00<\x00@\x007\x00:\x00>\x00A\x005\x008\x00N\x00S\x00G\x00L\x00E\x00<\x00N\x00J\x00L\x003\x008\x00C\x00C\x00:\x00<\x00E\x005\x00H\x00<\x00.\x008\x000\x003\x00.\x005\x00\x1a\x00<\x003\x008\x00(\x00'\x00@\x007\x00>\x00<\x00>\x00E\x00E\x00J\x00J\x00<\x00E\x00P\x00N\x00E\x00Q\x00L\x00A\x00@\x00G\x00@\x00C\x00@\x00C\x00G\x003\x00E\x00>\x00L\x00U\x00L\x00L\x00X\x00H\x00W\x00P\x00S\x00H\x00Q\x00G\x00S\x00A\x00L\x00H\x00@\x00C\x00>\x00A\x00E\x00E\x00>\x00A\x00<\x00C\x00A\x00L\x00S\x00P\x00N\x00Z\x00Q\x00L\x00P\x00J\x00@\x00N\x00G\x00P\x00P\x00a\x00`\x00l\x00`\x00a\x00P\x00Q\x00P\x00Q\x00P\x00N\x00N\x007\x00Q\x00e\x00c\x00X\x00C\x00@\x00U\x00L\x00J\x00n\x00`\x00^\x00S\x00P\x00^\x00^\x00H\x00H\x00G\x00<\x00E\x007\x00:\x00:\x005\x00,\x00A\x00>\x001\x00>\x003\x00<\x00@\x00:\x00N\x00@\x00S\x00Q\x00H\x00J\x00W\x00<\x00P\x00X\x00L\x00U\x00S\x00J\x00Q\x00P\x00A\x00P\x008\x001\x00>\x00@\x00A\x00J\x001\x00*\x00A\x001\x00@\x00%\x00.\x00%\x00%\x00\x1e\x000\x00(\x00\x18\x00!\x00*\x00<\x001\x003\x003\x00@\x00E\x00<\x00@\x000\x00,\x000\x00\x1e\x00\x1e\x00\x18\x00\x13\x00\x0c\x00 \x00\x15\x00,\x001\x00\x1c\x00%\x00#\x00(\x00*\x00!\x001\x005\x007\x008\x005\x00:\x00*\x00C\x00.\x00:\x00*\x00,\x00\x1e\x00\x11\x00\x0e\x00\x13\x00\x0c\x00\x0c\x00\x1e\x00\x1c\x00\x11\x00\x1a\x00%\x00\x1e\x00\x1e\x00!\x00 \x00%\x00*\x00\x0c\x00\x17\x00\x11\x00\x05\x00\n\x00\x13\x00\x08\x00\x13\x00\x11\x00\n\x00\x0c\x00\x10\x00\x00\x00\xfa\xff\xf8\xff\xf7\xff\xf5\xff\xef\xff\xec\xff\xef\xff\xea\xff\xea\xff\xfa\xff\xf7\xff\xfe\xff\xf3\xff\xf1\xff\xee\xff\xf5\xff\xfc\xff\xf5\xff\xec\xff\xf5\xff\xef\xff\xf1\xff\xf1\xff\xec\xff\xf5\xff\xf5\xff\xef\xff\xf7\xff\xf8\xff\xf1\xff\xef\xff\xf3\xff\xf8\xff\xf3\xff\xf5\xff\xe5\xff\xf8\xff\xfc\xff\x05\x00\xf8\xff\xfa\xff\x00\x00\xfc\xff\xee\xff\xf3\xff\xf5\xff\xfa\xff\xf1\xff\xef\xff\xdf\xff\x05\x00\xe1\xff\xdc\xff\xec\xff\xe5\xff\xe7\xff\xec\xff\xe3\xff\xe3\xff\xd8\xff\xdf\xff\xf5\xff\xf8\xff\xea\xff\xef\xff\xfc\xff\x00\x00\xfc\xff\x1c\x00\x1c\x00\x0e\x00\x1c\x00\x10\x00\x17\x00\x05\x00\x18\x00\x17\x00\x0e\x00\x0e\x00\x11\x00\x11\x00\x03\x00\n\x00\x01\x00\x0e\x00\n\x00\n\x00\x13\x00\x10\x00\x13\x00\x11\x00\x0c\x00\x03\x00\x05\x00\xfe\xff\xef\xff\xf5\xff\xe3\xff\xda\xff\xd5\xff\xdf\xff\xda\xff\xe1\xff\xe8\xff\xe8\xff\xe1\xff\xde\xff\xd3\xff\xcf\xff\xcc\xff\xcc\xff\xe8\xff\xe8\xff\xde\xff\xdf\xff\xea\xff\xde\xff\xe7\xff\xdf\xff\xea\xff\xe1\xff\xe7\xff\xf1\xff\xec\xff\xf5\xff\xf5\xff\xf1\xff\xec\xff\xf3\xff\xe7\xff\xdf\xff\xe5\xff\xf7\xff\xf5\xff\xea\xff\xec\xff\xdc\xff\xd5\xff\xd5\xff\xd8\xff\xd1\xff\xd5\xff\xdc\xff\xdf\xff\xe8\xff\xe7\xff\xf1\xff\xec\xff\xec\xff\xee\xff\xf5\xff\xf8\xff\xe8\xff\xf1\xff\xe1\xff\xea\xff\xdf\xff\xea\xff\xef\xff\xfa\xff\x0c\x00\x03\x00\xf8\xff\x05\x00\x01\x00\xfe\xff\xf8\xff\xfe\xff\xfa\xff\x03\x00\x08\x00\x03\x00\x05\x00\x03\x00\xfc\xff\xfc\xff\xf7\xff\xf8\xff\xfa\xff\x05\x00\x01\x00\xf7\xff\xf1\xff\xef\xff\xea\xff\xec\xff\xf1\xff\xea\xff\xea\xff\xe5\xff\xea\xff\xdf\xff\xef\xff\xf1\xff\xef\xff\xf5\xff\xf8\xff\xf1\xff\xea\xff\xf3\xff\xf7\xff\xec\xff\xe8\xff\xe7\xff\xec\xff\xde\xff\xd3\xff\xcf\xff\xce\xff\xcc\xff\xd5\xff\xca\xff\xca\xff\xd5\xff\xdc\xff\xe3\xff\xec\xff\xef\xff\xf1\xff\x00\x00\xf7\xff\xf5\xff\xf3\xff\xf8\xff\xee\xff\xf3\xff\xfa\xff\xdf\xff\xe8\xff\xf3\xff\x01\x00\x01\x00\xf7\xff\xec\xff\xfa\xff\xf1\xff\x01\x00\xf8\xff\xf8\xff\xf3\xff\xf1\xff\xf5\xff\xfa\xff\x00\x00\xf8\xff\x08\x00\xfc\xff\xe7\xff\xef\xff\xfa\xff\xfe\xff\xee\xff\xfc\xff\xf3\xff\xee\xff\xd7\xff\xde\xff\xd7\xff\xd3\xff\xcf\xff\xbe\xff\xcf\xff\xcf\xff\xdf\xff\xcf\xff\xde\xff\xd5\xff\xce\xff\xce\xff\xe1\xff\xcf\xff\xe5\xff\xda\xff\xc8\xff\xce\xff\xc7\xff\xc1\xff\xc7\xff\xbe\xff\xca\xff\xc1\xff\xbf\xff\xc7\xff\xd1\xff\xcf\xff\xd3\xff\xdf\xff\xea\xff\xe5\xff\xdc\xff\xdc\xff\xe1\xff\xd7\xff\xcc\xff\xcf\xff\xd3\xff\xc1\xff\xc3\xff\xc8\xff\xd3\xff\xdc\xff\xde\xff\xe1\xff\xf5\xff\xd1\xff\xde\xff\xd8\xff\xda\xff\xd7\xff\xde\xff\xdf\xff\xde\xff\xde\xff\xe8\xff\xda\xff\xd5\xff\xd8\xff\xd8\xff\xd5\xff\xd1\xff\xca\xff\xcc\xff\xd7\xff\xde\xff\xe3\xff\xe7\xff\xec\xff\xde\xff\xe7\xff\xe5\xff\xe1\xff\xda\xff\xdf\xff\xde\xff\xf5\xff\xf1\xff\xf7\xff\xfe\xff\xf8\xff\x01\x00\xf8\xff\x00\x00\xfa\xff\x00\x00\x03\x00\x01\x00\x00\x00\xf5\xff\xf3\xff\xef\xff\xf5\xff\xef\xff\xe8\xff\xf7\xff\xea\xff\xdf\xff\xe1\xff\xe5\xff\xdf\xff\xd8\xff\xcf\xff\xd3\xff\xd1\xff\xda\xff\xd7\xff\xdf\xff\xe7\xff\xda\xff\xd7\xff\xd1\xff\xd3\xff\xd5\xff\xce\xff\xd3\xff\xe1\xff\xe7\xff\xde\xff\xce\xff\xcc\xff\xc8\xff\xcc\xff\xd8\xff\xe5\xff\xd3\xff\xdf\xff\xdf\xff\xef\xff\xc7\xff\xcf\xff\xd8\xff\xd3\xff\xc8\xff\xd8\xff\xd3\xff\xcf\xff\xd5\xff\xd5\xff\xd7\xff\xe1\xff\xd8\xff\xda\xff\xd1\xff\xd3\xff\xec\xff\xe7\xff\xb1\xff\xcc\xff\xcf\xff\xba\xff\xba\xff\xcc\xff\xaf\xff\xb3\xff\xc8\xff\xbe\xff\xcc\xff\xb8\xff\xbe\xff\xc5\xff\xc5\xff\xb5\xff\xb8\xff\xb3\xff\xbf\xff\xc1\xff\xbf\xff\xbf\xff\xae\xff\xb8\xff\xbf\xff\xb5\xff\xb5\xff\xb1\xff\x91\xff\xaa\xff\xa3\xff\xae\xff\xa7\xff\xa8\xff\xa3\xff\xac\xff\xa7\xff\xaa\xff\xa8\xff\xb5\xff\x98\xff\xac\xff\xa1\xff\x95\xff\xa5\xff\xaa\xff\xa1\xff\xa7\xff\x9e\xff\xa1\xff\xa3\xff\xb1\xff\xb8\xff\xaa\xff\xac\xff\xac\xff\xb5\xff\xb3\xff\xc1\xff\xb1\xff\xae\xff\xac\xff"
[5]:
len(bit_data) #original chunksize is 4096
[5]:
8192
Unpack bit data#
[6]:
import struct
data_int = struct.unpack(f"{CHUNK_SIZE}h",bit_data) #h for signed integer of 2 bytes(16 bits)
[25]:
np.frombuffer(bit_data, dtype = np.int16)
[25]:
array([-22, -18, -33, ..., -61, -59, -66], dtype=int16)
Plot data#
[7]:
%matplotlib inline
fig, ax = plt.subplots()
ax.plot(data_int)
plt.show()
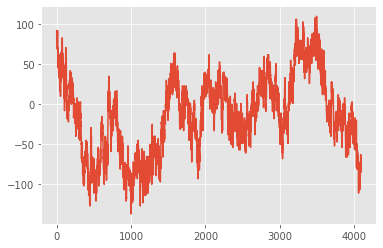
Live audio setup#
Live in tkinter#
%matplotlib tk
will open tkinter window for better live
[28]:
import numpy as np
import pyaudio
import struct
import matplotlib.pyplot as plt
from matplotlib import style
style.use('ggplot')
%matplotlib tk
CHUNK_SIZE = 1024 * 4 # how many audio samples per frame
FORMAT = pyaudio.paInt16 # 16 bit audio format in bits
CHANNELS = 1
RATE = 44100 # samples per second : 44.1 KHz
# Port audio interface on Pyaudio
p = pyaudio.PyAudio()
stream = p.open(
format=FORMAT,
channels=CHANNELS,
rate=RATE,
input=True,
output=True,
frames_per_buffer=CHUNK_SIZE
)
fig, ax = plt.subplots()
ax.set_ylim(-2**15 ,2**15)
line, = ax.plot(range(CHUNK_SIZE),range(CHUNK_SIZE))
while True:
try:
bit_data = stream.read(CHUNK_SIZE)
data_int = np.frombuffer(bit_data, dtype = np.int16) #h for signed integer of 2 bytes(16 bits)
line.set_ydata(data_int)
fig.canvas.draw()
fig.canvas.flush_events()
except Exception as e:
print(e)
break
invalid command name "pyimage130"
Live in browser#
[40]:
import numpy as np
import pyaudio
import struct
import matplotlib.pyplot as plt
from matplotlib import style
from matplotlib import animation
from IPython import display
style.use('ggplot')
%matplotlib inline
fig, ax = plt.subplots()
ax.set_ylim(-2**15 ,2**15)
line, = ax.plot(range(CHUNK_SIZE),range(CHUNK_SIZE))
def draw_frame(n):
bit_data = stream.read(CHUNK_SIZE)
data_int = np.frombuffer(bit_data, dtype = np.int16) #h for signed integer of 2 bytes(16 bits)
line.set_ydata(data_int)
return (line,)
anim = animation.FuncAnimation(fig, draw_frame, frames=500, interval=100, blit=True)
display.HTML(anim.to_html5_video())
[40]:
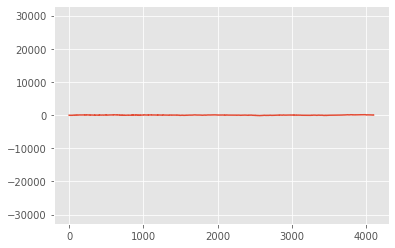
FFT processing & Power Spectral Density#
Live in tkinter#
[3]:
import numpy as np
import pyaudio
import struct
import matplotlib.pyplot as plt
from matplotlib import style
style.use('dark_background')
%matplotlib tk
CHUNK_SIZE = 1024 * 4 # how many audio samples per frame
FORMAT = pyaudio.paInt16 # 16 bit audio format in bits
CHANNELS = 1
RATE = 44100 # samples per second : 44.1 KHz
# Port audio interface on Pyaudio
p = pyaudio.PyAudio()
stream = p.open(
format=FORMAT,
channels=CHANNELS,
rate=RATE,
input=True,
output=True,
frames_per_buffer=CHUNK_SIZE
)
fig = plt.figure(figsize=(15,15))
ax1 = fig.add_subplot(2,2,(1,2))
ax2 = fig.add_subplot(2,2,(3,4))
ax1.set_ylim(-2**15 ,2**15)
ax2.set_ylim(0,1e10)
ax1.set_title('Audio Signal')
ax2.set_title('Power Spectral Density')
n = int(np.floor(CHUNK_SIZE/2))
line1, = ax1.plot(range(CHUNK_SIZE),range(CHUNK_SIZE))
line2, = ax2.plot(range(n),range(n))
while True:
try:
bit_data = stream.read(CHUNK_SIZE)
data_int = np.frombuffer(bit_data, dtype = np.int16) #h for signed integer of 2 bytes(16 bits)
f_hat = np.fft.fft(data_int,CHUNK_SIZE)
psd = (f_hat * np.conjugate(f_hat) / CHUNK_SIZE).real
line1.set_ydata(data_int)
line2.set_ydata(psd[:n])
fig.canvas.draw()
fig.canvas.flush_events()
except Exception as e:
print(e)
break
invalid command name "pyimage11"
Live in browser#
[6]:
import numpy as np
import pyaudio
import struct
import matplotlib.pyplot as plt
from matplotlib import style
from matplotlib import animation
from IPython import display
style.use('ggplot')
%matplotlib inline
CHUNK_SIZE = 1024 * 4 # how many audio samples per frame
FORMAT = pyaudio.paInt16 # 16 bit audio format in bits
CHANNELS = 1
RATE = 44100 # samples per second : 44.1 KHz
# Port audio interface on Pyaudio
p = pyaudio.PyAudio()
stream = p.open(
format=FORMAT,
channels=CHANNELS,
rate=RATE,
input=True,
output=True,
frames_per_buffer=CHUNK_SIZE
)
fig = plt.figure(figsize=(10,10))
ax1 = fig.add_subplot(2,2,(1,2))
ax2 = fig.add_subplot(2,2,(3,4))
ax1.set_ylim(-2**15 ,2**15)
ax2.set_ylim(0,1e10)
ax1.set_title('Audio Signal')
ax2.set_title('Power Spectral Density')
m = int(np.floor(CHUNK_SIZE/2))
line1, = ax1.plot(range(CHUNK_SIZE),range(CHUNK_SIZE))
line2, = ax2.plot(range(m),range(m))
def draw_frame(n):
bit_data = stream.read(CHUNK_SIZE)
data_int = np.frombuffer(bit_data, dtype = np.int16) #h for signed integer of 2 bytes(16 bits)
f_hat = np.fft.fft(data_int,CHUNK_SIZE)
psd = (f_hat * np.conjugate(f_hat) / CHUNK_SIZE).real
line1.set_ydata(data_int)
line2.set_ydata(psd[:m])
return (line1,line2)
anim = animation.FuncAnimation(fig, draw_frame, frames=200, interval=100, blit=True)
display.HTML(anim.to_html5_video())
[6]:
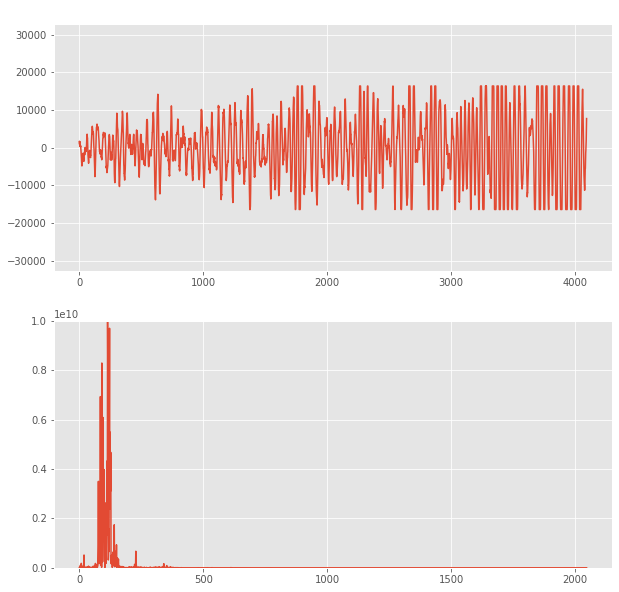